Prerequisites:
- Visual Studio Code (VS Code)
- An AWS Builder ID or…
- Complete the 2-step Amazon Q Developer in the IDE section in the Amazon Q Getting Started guide. It’s about 4-ish clicks and an e-mail authorization.
Beginner’s Q in Practice
If you’re just starting out with coding or exploring Amazon Web Services (AWS), Amazon Q Developer is a great tool to have in your corner. Integrated right into Visual Studio Code (VS Code) and other IDEs, it’s like having a coding tutor who’s also an AWS expert. In this post, I’ll walk you through three beginner-friendly use-cases that show off what Amazon Q can do.
There are so many great AI tools out there that it’s sometimes hard to know which to use! It’s not a matter of which is “best,” but it’s really about which one best fits the use-case. In the following 3 beginner use-cases, I’ll highlight why Q stands out compared to other AI tools like ChatGPT or Grok.
Let’s get to building!
Use-Case 1. Learning AWS Basics Through Guided Exploration
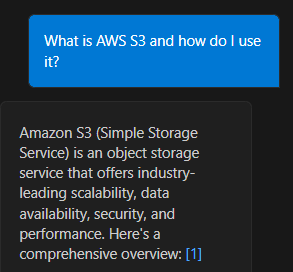
New to AWS? No problem. Amazon Q Developer can help you get the hang of it, starting with the basics. Imagine you’re curious about AWS S3, a popular cloud storage service. Open VS Code, bring up Amazon Q, and simply ask: “What is AWS S3 and how do I use it?”
Amazon Q will explain that S3 is like a giant online filing cabinet for storing files. And it’s likely to give you a simple Python script to upload a file using the boto3 library. That’s the python library for working with AWS. Here’s the code I got in response:
#python
import boto3
s3 = boto3.client('s3')
bucket_name = 'my-first-bucket'
file_name = 'hello.txt'
s3.upload_file(file_name, bucket_name, file_name)
print(f"Uploaded {file_name} to {bucket_name}!")
Not bad at all! Not only does it give you code, it’ll even break down each line, like how boto3.client(‘s3’) connects to S3 and upload_file does the heavy lifting. To try this script out, you’ll need AWS credentials set up (Q can guide you there too… just ask!). Save a hello.txt
file, change the bucket name value, and run it. And voila! …with Q’s help, you went from 0 to uploading in minutes!
You’re a pro already and you don’t even know it!
Why Amazon Q Shines Here
Unlike ChatGPT or Grok, Amazon Q is built with AWS in mind; the AI model is trained on it! It doesn’t just give generic answers, it ties explanations to AWS services and hands you working code tailored to them. ChatGPT might guess or give outdated AWS info, and Grok, while smart, isn’t as focused on Amazon’s ecosystem. For beginners, Q’s AWS expertise can save you a ton of time stuck in “tutorial hell” or digging around Stack Overflow just hoping someone saw your issue before.
Use-Case 2. Generating Starter Code for a Basic Static Website
Ready to build something? Let’s make a simple website with Amazon Q. In VS Code, ask: “Give me a single-file website with a heading, a styled button, and a click counter.” The result we want here is a single HTML file that includes CSS and Javascript. We’ll refactor in Use-Case 3.
Amazon Q whips up something like this in seconds. I say “like this” because AI just works that way often giving unique output with subtle differences:
<html>
<head>
<style>
body { font-family: Arial, sans-serif; text-align: center; }
button { background-color: #4CAF50; color: white; padding: 10px 20px; border: none; cursor: pointer; }
button:hover { background-color: #45a049; }
</style>
</head>
<body>
<h1>Welcome to My Site. I love AI and the Dagley Blog!</h1>
<button onclick="countClicks()">Click Me!</button>
<p>Clicks: <span id="counter">0</span></p>
<script>
let clicks = 0;
function countClicks() {
clicks += 1;
document.getElementById('counter').innerText = clicks;
}
</script>
</body>
</html>
Save it as index.html, open it in a browser, and click the button. The counter ticks up! It’s got HTML for structure, CSS in the <style> tags for a green button, and JavaScript in <script> to track clicks.
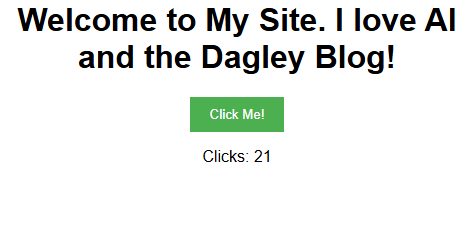
Feeling spicy? Want a blue button? Change #4CAF50 to #1E90FF and see it update.
Why Amazon Q Beats the Competition
Amazon Q integrates seamlessly with VS Code, so you’re chatting and coding in one place. ChatGPT can generate code, but you’d need to copy-paste it into your editor, and it might not catch your project’s context. Grok’s great for general questions, but Q’s ability to churn out practical, beginner-ready code right in your workflow is smoother and faster.
Use-Case 3. Refactoring Code into Separate HTML, CSS, and JS Files
Now that you’ve got a working site, let’s make it neater by refactoring. This means, we’ll clean up the code, but not change the the function and output of the code. Ask Amazon Q: “Split this into separate HTML, CSS, and JS files.” It’ll refactor that single file into three:
index.html:
<html>
<head>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1>Welcome to My Site. I love AI and the Dagley Blog!</h1>
<button onclick="countClicks()">Click Me!</button>
<p>Clicks: <span id="counter">0</span></p>
<script src="script.js"></script>
</body>
</html>
styles.css:
body {
font-family: Arial, sans-serif;
text-align: center;
}
button {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
script.js:
let clicks = 0;
function countClicks() {
clicks += 1;
document.getElementById('counter').innerText = clicks;
}
Save these in the same folder, open index.html in a browser, and it still works! The HTML links to styles.css with <link> and script.js with <script>. This setup is cleaner and closer to how pros organize code.
Amazon Q’s Edge
Amazon Q understands your code’s context in VS Code and refactors it on the fly. ChatGPT might split files but miss linking them properly, and Grok, while clever, isn’t as tuned for in-editor tasks. Q’s ability to refine your work without leaving VS Code keeps things simple for beginners and makes Q the clear winner for code assistance.
Why Start with Amazon Q in VS Code?
These use-cases build on each other. First, you learn AWS basics to get comfy with the cloud. Then, you generate a quick website to see results fast. Finally, you clean it up to level up your skills. Amazon Q makes it all painless with clear answers and instant code, right where you’re working.
While some seasoned engineers might argue that you need to learn the fundamentals, Q is still a great partner in your education. You can even ask it questions that don’t make any sense, and Q will still try. For example, “How to a unfreeze an EC2 instance in Glacier?” It doesn’t make any sense, but Q will teach you about EC2 instances, Glacier, and then it will make some attempt to guess what you’re attempting and provide a path to solution.
Compared to ChatGPT, Q’s AWS focus and VS Code integration save you time and guesswork. Unlike Grok, it’s less about broad chats and more about actionable coding help. For beginners, that targeted support, tied to Amazon’s tools, is pure gold. Ready to try it? Fire up VS Code, summon Q, and start with “What is AWS S3?” You’ll be amazed at how far you get!
Cue the Q!
-Ryan